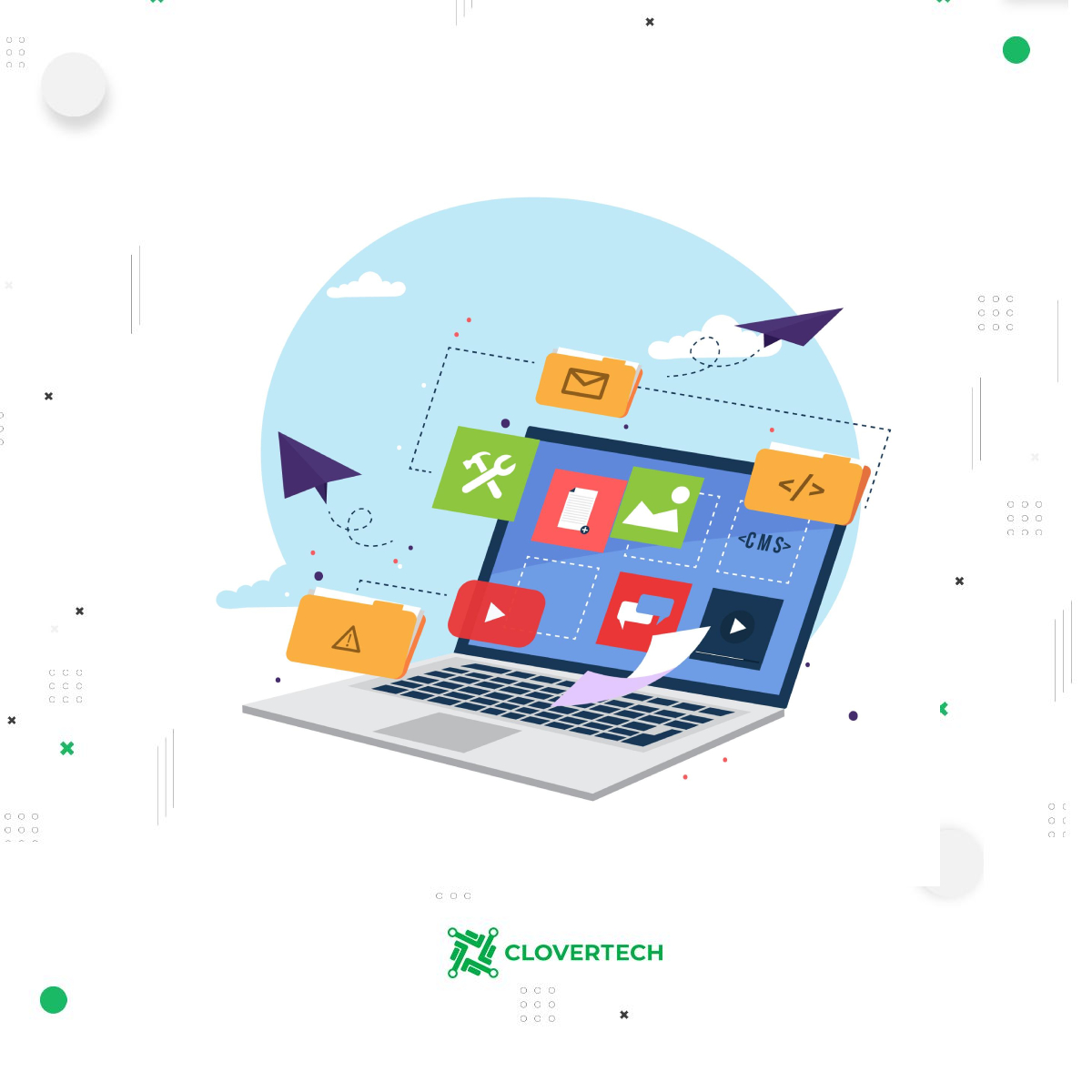
Web Development: The Static Basics
This article aims to clarify the basics of static web development. Namely, we will focus on the implementation processes next time.
Generally, a website does not differ from an application much. The only difference between those is that the website’s functionality is limited by the browser possibilities. In other words, any website is something that can be read by web browsers only.
There are two main divisions of web development, the front-end, and the back-end sides. Let’s consider the front-end first.
Frontend Web
Any website is a web pages collections that, in their turn, are simple HTML files. When you send a request to a website’s server, the browser receives a lot of data in the response. And the thing that ensures all of that is HTML.
Every web page is a separate HTML file. Anytime we go to another route, the browser makes a new request to get the HTML file for the required page. For instance, if you go from /home to /about-me, it sends a request to the server to get the about-me’s HTML.
The best way to check that is to pay attention to the loading indicator in your browser. If it displays that the site is being loaded, that means you have sent a request to the server, and the browser is waiting for the response.
Definition: HTML stands for HyperText Markup Language, where hypertext refers to a text that links to some other text. Essentially, that is the way you go from one page to another.
Do you like this button’s appearance? (Click Run Pen to preview)
Well, you most likely don’t. And what about this one?
The first one is a simple HTML button with default styles. The second one uses CSS to add personalized styles. The following is an example of the second button’s code:
<!-- HTML --> <button>I'm a Fancy Button!</button>/* CSS */ button { color: value; /* consider 'value' as a placeholder */ background: value; border: value; padding: value; border-radius: value; box-shadow: value; }
Definition: CSS stands for Cascading Style Sheets. The styles are applied from the top to the bottom, in the defined order, that forms something like cascading waterfall.
Any repeated style overrides its previous corresponding values. For example:
button { color: white; } /* This will override the previous value for 'color' */ button { color: black; }
JavaScript
HTML and CSS aren’t enough to create a modern website. You may have noticed that the example button we’ve listed above does nothing when it’s clicked, however, it is meant to trigger an action.
Click on the following example to see the difference.
Fancy Button With Interaction (Blog Demo)
JavaScript adds functionality to these previews. Same as HTML and CSS, JavaScript is a client-side programming language. Namely, it can run only inside a web browser, unlike C++, Java, Python, and other popular server-side programming languages.
Working in JavaScript is similar to many other programming languages. For instance, the following statement declares a variable named myName
, and we can do anything with it.
var myName = 'Sapinder Singh';
We can use this variable upon demand. However, we want to work with HTML elements too. So how do we work with those in JavaScript?
You may have heard about API (which stands for Application Programming Interface). API serves as an interface for various program products to ensure the interaction and data sharing between those.
To interact with HTML, JavaScript uses DOM API (DOM stands for Document Object Model). It provides an HTML document to JavaScript as an objected model. The root of the inverted tree-like object is the document itself. Each element of the document gets its own node that’s depth depends on the number of children elements it has.
Thus, to select the “Fancy Button” in JavaScript with DOM API, it is required to do the following:
// assume that the button does have an ID var fancyButton = document.getElementById("fancy-button");
Instead of assigning a common value to a variable, it is required to assign a DOM element to it. After that, the variable may be considered as an actual page element. The following statement implements that ‘click’ action to the button:
/* Don't worry if you don't understand the syntax, you're here just to understand what it does. */ fancyButton.onclick = () => alert("I've been clicked! :)");
Namely, HTML, CSS, and JavaScript are the basis of the front-end of any modern website. It opens several opportunities for us:
-
it is possible to have one or several HTML files (and additional files like .css, .js., etc. that are bound inside an HTML);
-
open the root HTML file in any web browser;
-
see it is being displayed as a web page.
As you may have guessed, all of these are quite useless unless we can only see the web pages. That is why we have to discuss back-end development.
Webserver
A web server is meant to process our pages all over the world. We have several programming languages for this task: Java, PHP, Ruby, Python, C++, and many others. We have mentioned above that JavaScript is not a back-end programming language. Well, it’s been kind of a lie!
Meet Node.js
Node.js is a JavaScript runtime environment. It combines various tools and technologies that help provide a suitable environment to enable it to run a certain program or application.
Your browser also provides a JavaScript runtime environment that supplies various APIs for the code JavaScript engine, f.e.:
- DOM for parsing HTML;
- API storage for reserving a website’s data on the client’s system;
- CSSOM for managing styles using JavaScript.
However, the core part of a JavaScript runtime environment is the JavaScript engine.
Google Chrome and other web browsers that are based on Chromium use Google V8 to launch JavaScript, which is written in C++. Node.js uses the same engine. However, instead of providing APIs like DOM or others, it uses other tools to allow accessing OS, File System, Networking, etc. As a result, this enables using JavaScript as a server-side programming language.
Node.js is widely used for server development. Its main advantage is that one doesn’t have to learn another language to create a server. Knowing JavaScript is fair enough for that.
Server Work
If a server is not crashed, it’s always up and running. It receives the requests and sends the proper responses to the clients. The server’s response type depends on the type of request the user makes. These are called methods. The two most widely used request methods over HTTP are:
- GET is a method that is better to be used for retrieving a resource from the server. When you go to any website, the browser you use sends the GET request to the server asking to “display” the required page in return.
- Relational/SQL databases store data in a table-like format. In such tables, each row represents an entity, and each column provides certain data about that entity such as Name, Address, etc. MySQL and PostgreSQL are two popular relational databases when it comes to working with Node.js.
- Non-Relational/NoSQL databases are more flexible than SQL ones since the “No” in NoSQL stands for “not only”. The most popular NoSQL choices with Node.js are MongoDB and Redis.
POST is a method that should be used for submitting data to the server, usually with creating a new resource. When you fill out the webform, it mostly uses the POST method, for instance:
<!-- HTML --> <form method="POST"> <!-- The form fields go here --> </form>
Since the actual implementation of how a server handles requests and responses in Node.js might be a little complex for beginners, we will use the Pseudocode to show it:
CREATE an http server using http.createServer() CALL server.listen() to activate the server // Now handle requests from a different path, e.g. '/home' or any other path IF request.url EQUALS '/home' SEND '/home.html' ELSE IF request.url EQUALS '/create-user' // the user wants to visit the page where they are able to create a new account IF request.method EQUALS 'GET' SEND '/create-user.html' // if the method is POST, it means the user submitted a form on '/create-user' path ELSE IF request.method EQUALS 'POST' SEND newlyCreatedAccount
Now that we have an overview of what a server is, you may have noticed the POST method handler on the /create-user path. That is how we create a new user based on the data received via request object, and then provide that newlyCreateAccount to the user. But now we have to save and store this account to remember it in the future. It’s time to discuss databases.
Databases
You probably already have an idea of what a database is. A database is a peculiar data storage with a certain data organizing and processing structure. A common term used relating to databases is CRUD. The abbreviation stands for Create, Read, Update, and Delete. These are the most basic processes performed by a database.
There are various types of databases, however, there are two major categories: Relational and Non-Relational; sometimes they are referred to as SQL (which stands for Structured Query Language) and NoSQL respectively. Let’s take a brief look at each of these:
- Relational/SQL databases store data in a table-like format. In such tables, each row represents an entity, and each column provides certain data about that entity such as Name, Address, etc. MySQL and PostgreSQL are two popular relational databases when it comes to working with Node.js.
- Non-Relational/NoSQL databases are more flexible than SQL ones since the “No” in NoSQL stands for “not only”. The most popular NoSQL choices with Node.js are MongoDB and Redis.
When it comes to choosing a database, the choice must depend on the type of data you want to store.
It is recommended to learn about various options and choose the most optimal one to get better performance.
Server-side APIs
Have you ever thought about how the weather application in your own phone gathers that much weather information? And how do Google Maps detect your location? And where does this rest countries application get all the data from?
It is all performed using the APIs. A server API is similar to web servers; however, instead of serving a web application, it serves data to other applications so these apps are able to use it. The mentioned above rest countries application uses Rest Countries API to retrieve the data about all the countries. Visiting this endpoint you will get a lot of data instead of an HTML page.
An endpoint can be considered as a destination. We can visit it to get some data or perform any other action. For instance, the mentioned above API offers various endpoints that are to get different data fragments. To see what we mean, visit the following endpoints that will provide you the data of certain countries:
A server API can store any number of endpoints. Meanwhile, the API must correspond to a well-designed architecture to be maintainable and scalable for a large project. The standard protocol for web APIs is to use a RESTful architecture.
Let’s summarize the things we’ve learned so far:
- HTML, CSS, and JavaScript work together on the client-side.
- A server processes the requests and responses of the clients using Node.js.
- A data is stored in databases.
- API may serve instead of an application.
Now it’s time to go to the next part.
Hosting providers and domains
The so-called webserver we have learned about before is on our local machine only. We can run the server in the background and open it in a web browser, f.e. at the localhost:8000 to access our website. 8000 is a port number. However, if you want your server to be available all over the world, you must host it not on your machine locally, but somewhere else. In such a case, you must deploy the application to a hosting provider that is to launch your server on its huge machines 24/7.
A domain name is required for people to access your server on the Internet since it is not possible to reach it with the local host anymore. The domain name is the *****. com part of a URL. The domains may also have sub-domains.
An Interesting Fact
You can have any number of sub-domains when you own a domain. Moreover, the www. is a sub-domain, too!
Apart from domain purchases, you also have to pay the regular charges to the hosting provider since they support your server 24/7. However, many hosting providers offer free services with limited resources, and it’s possible to upgrade your account for extended requirements. The free resources that offer free domains are a great start! The main caution is that those domains end with the resources’ default domain names. For example, *.herokuapp.com for Heroku, *.netlify.app for Netlify, and so on. Thus, you’d have to buy your own domain name to look actually professional.
Version control
Supporting your code is crucial since it is the only way to detect and fix the current bugs, and add the new features to the application, too. Version Control Systems (VCS) allow tracking and adding changes. They are even capable of reverting the entire application back to its previous version!
The most widely used VC system is GIT. Here are a few terms you must know for using Git:
-
Branch. Branching allows developers to divide their code repositories into separate branches. Each branch has a specific purpose (for instance, you can maintain development and main branches). The number of branches may increase when the project enlarges.
-
Stage. Git provides a staging area, where all edits that are ready for implementation are stored. Adding the changed files in the code to the staging area via the
git add <file-name>
command allows us to able to review the changes before making a final commit. -
Commit. After the changes are reviewed, we have to run the
git commit
command to create a new version in the git history for our code repository. -
Merge. We can merge changes committed to any branch with the other branch’s changes. For instance, if you committed changes to the
development
branch, and then tested these, you are now able tomerge
them into yourmain
branch to publish changes to the server. -
Remote repositories
If you somehow lose the local repository, you can clone it from your remote repository with the git history.
The remote repositories are sometimes public. These are known as open-source projects since their code is publically available to all developers of the community. For instance, Firefox, Node.js, VLC Player, and Linux are open-source projects, and anyone can contribute to these!
The two of the most popular cloud-based/remote repository platforms are GitHub and GitLab (the former is the leading solution). These are somewhat like Facebook for programmers where they can demonstrate and maintain their projects.